https://refactoring.guru/design-patterns/chain-of-responsibility
Chain of Responsibility
/ Design Patterns / Behavioral Patterns Chain of Responsibility Also known as: CoR, Chain of Command Intent Chain of Responsibility is a behavioral design pattern that lets you pass requests along a chain of handlers. Upon receiving a request, each handle
refactoring.guru
특정 메시지 혹은 이벤트 등을 관련 있는 모두에서 전달하며, 알아서 처리하게 하는 방식.
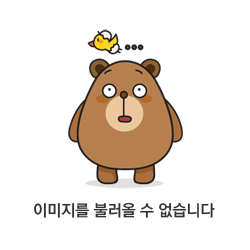
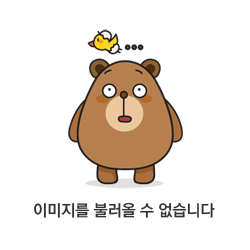
예제
- Dialog 등이 겹겹이 있을 때, device back button을 누를 때.
- Dialog component 안에서 색 변경을 눌러 관련 컴포넌트의 변화를 만들 때.
예제 코드
더보기
public abstract class ABackButtonHandler
{
protected ABackButtonHandler _nextHandler;
public void SetNextHandler(ABackButtonHandler next)
{
_nextHandler = next;
}
public abstract void OnBackButtonPressed();
}
public class MessageDialog : ABackButtonHandler
{
public override void OnBackButtonPressed()
{
if(_nextHandler != null)
{
_nextHandler.OnBackButtonPressed();
}
else
{
// DO OWN thing.. - close this dialog?
}
}
}
public class NormalDialog : ABackButtonHandler
{
public override void OnBackButtonPressed()
{
if(_nextHandler != null)
{
_nextHandler.OnBackButtonPressed();
}
else
{
// DO OWN thing.. - close this dialog ?
}
}
}
public class MainView : ABackButtonHandler
{
public override void OnBackButtonPressed()
{
if(_nextHandler != null)
{
_nextHandler.OnBackButtonPressed();
}
else
{
// DO OWN thing.. - show message? "Would you want to close the app?"
}
}
}
internal class ChainOfResponsibilityDemo
{
public void Run()
{
MainView _view = new MainView();
NormalDialog _dlg = new NormalDialog();
MessageDialog _msgDlg = new MessageDialog();
_view.SetNextHandler(_dlg);
_dlg.SetNextHandler(_msgDlg);
_view.OnBackButtonPressed();
}
}
'Work & Programming > System Design' 카테고리의 다른 글
[Behavioral Pattern] - Memento (0) | 2024.05.16 |
---|---|
[Behavioral Pattern] - Command (0) | 2024.05.16 |
[Structural Pattern] - Proxy (0) | 2024.05.15 |
[Structural Pattern] - Flyweight (0) | 2024.05.15 |
[Structural Pattern] - Facade (0) | 2024.05.15 |