https://leetcode.com/problems/longest-substring-without-repeating-characters/description/
문자열이 주어질 때, char의 중복이 없는 가장 긴 sub string의 길이를 구하라.
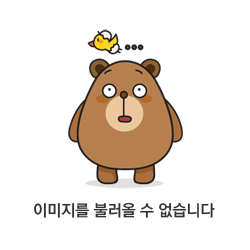
전형적인 sliding window 문제.
중복이 생기면 중복이 제거될 때까지 right를 당긴다.
코드
더보기
int lengthOfLongestSubstring(string s)
{
int left = 0, right = 0;
set<char> setBuff;
int maxLen = 0;
for (; right < s.length(); ++right)
{
char cur = s[right];
if (setBuff.find(cur) != setBuff.end())
{
while (left < s.length())
{
char chLeft = s[left];
setBuff.erase(chLeft);
++left;
if (chLeft == cur)
break;
}
}
maxLen = max(maxLen, right - left + 1);
setBuff.insert(cur);
}
return maxLen;
}
결과
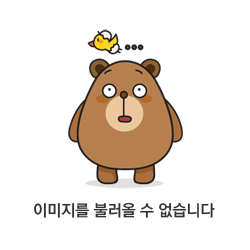
'Leetcode > NeetCode' 카테고리의 다른 글
[SlidingWindow][Medium] 567. Permutation in String (0) | 2024.07.10 |
---|---|
[SlidingWindow][Medium] 424. Longest Repeating Character Replacement (0) | 2024.07.10 |
[SlidingWindow][Easy] 121. Best Time to Buy and Sell Stock (0) | 2024.07.09 |
[TwoPointers][Medium] 11. Container With Most Water (0) | 2024.07.09 |
[TwoPointers][Medium] 15. 3Sum (0) | 2024.07.09 |